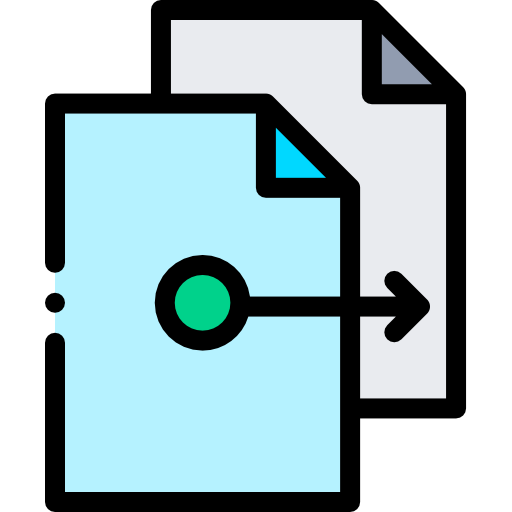
Практические решения для слияния
Комплексный подход к слиянию
Унифицированная структура слияния
граф TD
A[Input Files] –> B{Определение разделителей}
B –> C[Preprocessing]
C –> D[Transformation]
D –> E[Merge Process]
E –> F[Validated Output]
A[Input Files] –> B{Определение разделителей}
B –> C[Preprocessing]
C –> D[Transformation]
D –> E[Merge Process]
E –> F[Validated Output]
Техника решения слияния
1. Слияние на основе Python
import pandas as pd
def merge_mixed_delimiter_files(files):
dataframes = []
for file in files:
if file.endswith('.csv'):
df = pd.read_csv(file)
elif file.endswith('.tsv'):
df = pd.read_csv(file, sep='\t')
else:
df = pd.read_csv(file, sep='|')
dataframes.append(df)
merged_df = pd.concat(dataframes, ignore_index=True)
return merged_df
2. Слияние на основе сценариев Bash
#!/bin/bash
## Advanced file merge script
merge_files() {
local output_file=$1
shift
local input_files=("$@")
for file in "${input_files[@]}"; do
case "$file" in
*.csv)
csvtool col 1- "$file" >> "$output_file"
;;
*.tsv)
cat "$file" >> "$output_file"
;;
*)
awk '{print}' "$file" >> "$output_file"
;;
esac
done
}
merge_files output.txt file1.csv file2.tsv file3.txt
Сравнение стратегий слияния
Стратегия | Сложность | Гибкость | Производительность |
---|---|---|---|
Bash-скрипт | Низкий | Умеренный | Быстрый |
Python Pandas | Высокий | Очень высокий | Умеренный |
Обработка AWK | Средний | Высокий | Эффективный |
Дополнительные соображения по слиянию
Работа со сложными сценариями
- Обработка больших файлов
- Оптимизация памяти
- Обработка ошибок
- Настройка производительности
граф LR
A[Merge Input] –> B{Препроцессинг}
B –> C[Delimiter Normalization]
C –> D[Parallel Processing]
D –> E[Merge Execution]
E –> F[Output Validation]
A[Merge Input] –> B{Препроцессинг}
B –> C[Delimiter Normalization]
C –> D[Parallel Processing]
D –> E[Merge Execution]
E –> F[Output Validation]
Стратегии обработки ошибок
Надежный сценарий слияния
#!/bin/bash
## Robust file merge with error handling
merge_with_validation() {
local output_file=$1
shift
local input_files=("$@")
## Validate input files
for file in "${input_files[@]}"; do
if [[ ! -f "$file" ]]; then
echo "Error: File $file not found"
exit 1
fi
done
## Merge with error checking
if ! cat "${input_files[@]}" > "$output_file"; then
echo "Merge operation failed"
exit 1
fi
echo "Merge completed successfully"
}